Home
Mailgun Blog
Dev Life category
Verify Emails with Python and Mailgun API with this Step-by-Step Guide
Verifying emails using Python 3.9+ and the Mailgun API
Learn to integrate Mailgun’s Bulk Email Validation API with Python. This guide covers setup, secure API use, and efficient email list verification.
PUBLISHED ON
Whether you're sending transactional emails, newsletters, or promotional content, maintaining a clean and verified email list can significantly improve deliverability, prevent your messages from ending up in spam folders, and protect your domain's reputation. Invalid or mistyped email addresses can lead to higher bounce rates, resulting in penalties from email service providers and reduced engagement. In this guide you’ll learn how to verify your emails with the Mailgun API.
Table of contents
Table of contents
01What is Mailgun’s Bulk Email Validation API?
02Implementing bulk email validation with Python and the Mailgun API
03Setting up Mailgun for bulk email validation
04Setting up a project directory and dependencies
05Creating a Python project and configuration script
06Preparing your mailing list file
07Creating a bulk validation job
08Developing functions to check job status and download results
09Developing functions to check job status and download results
10Parsing and handling validation results
12Testing by submitting a bulk email validation job request
13Testing by fetching the status of the bulk email validation job
What is Mailgun’s Bulk Email Validation API?
Mailgun's Bulk Email Validation API is designed to make email verification both scalable and efficient. With it, developers can validate entire lists of email addresses, checking for common issues such as syntax errors, disposable emails, or addresses with inactive mail servers. The API provides detailed results for each email, making it easy to take the appropriate action.
In this tutorial, you'll learn how to integrate Mailgun's Bulk Email Validation API into your Python applications. From setting up your Mailgun account and securely handling credentials to writing code that validates emails and processes the results, this guide provides step-by-step instructions to efficiently verify large email lists.
Implementing bulk email validation with Python and the Mailgun API
Before you start working through this tutorial, make sure you have the following:
A basic understanding of Python programming
Python 3.9+
A Mailgun account; a paid account is required for this tutorial
Experience with application development based on Django REST framework
This tutorial uses the Windows operating system. While all of the instructions should also work for Linux and macOS, make sure to change the operating system path delimiters or syntax references as needed.
Setting up Mailgun for bulk email validation
To start, log in to your Mailgun account and navigate to the dashboard. Scroll down and select the API keys option:
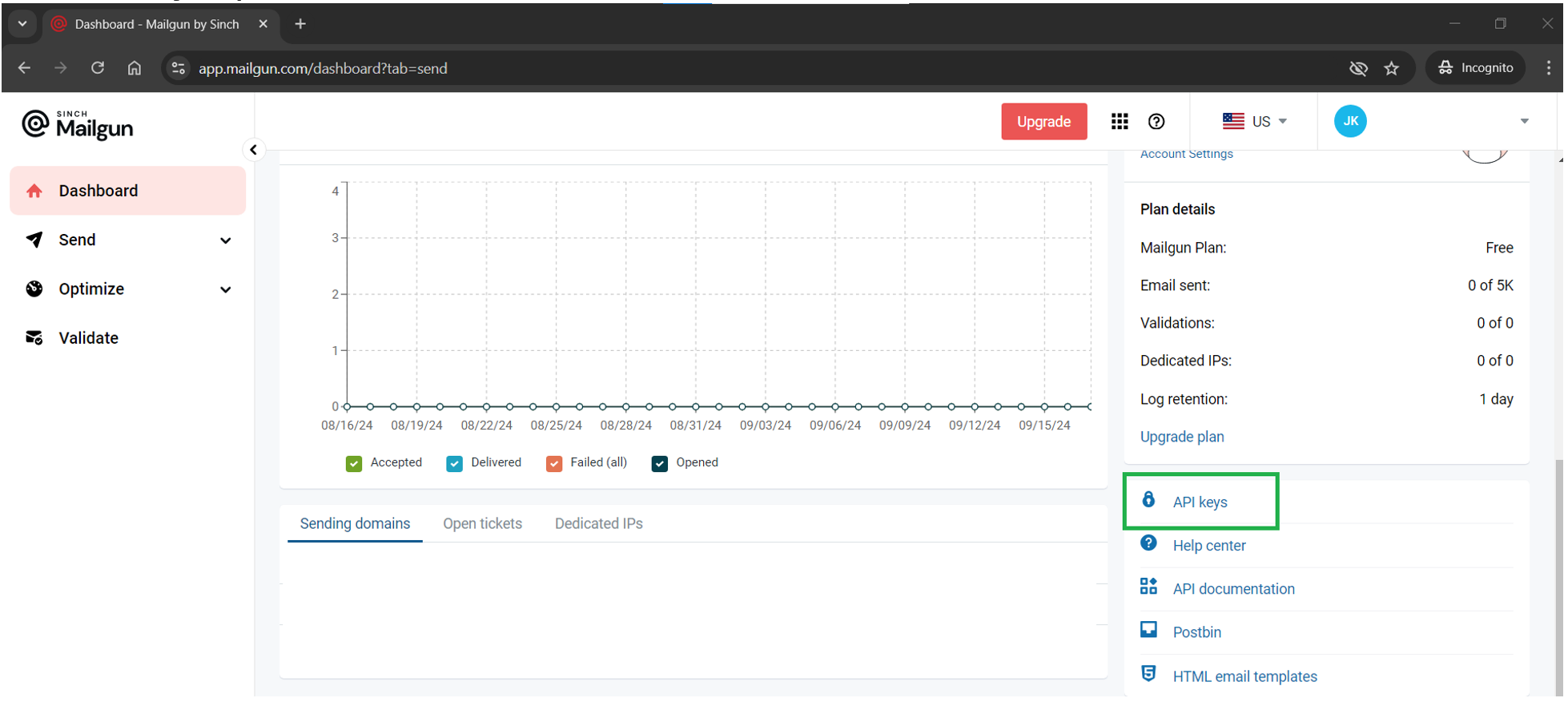
Click Add new key to add a new API key:
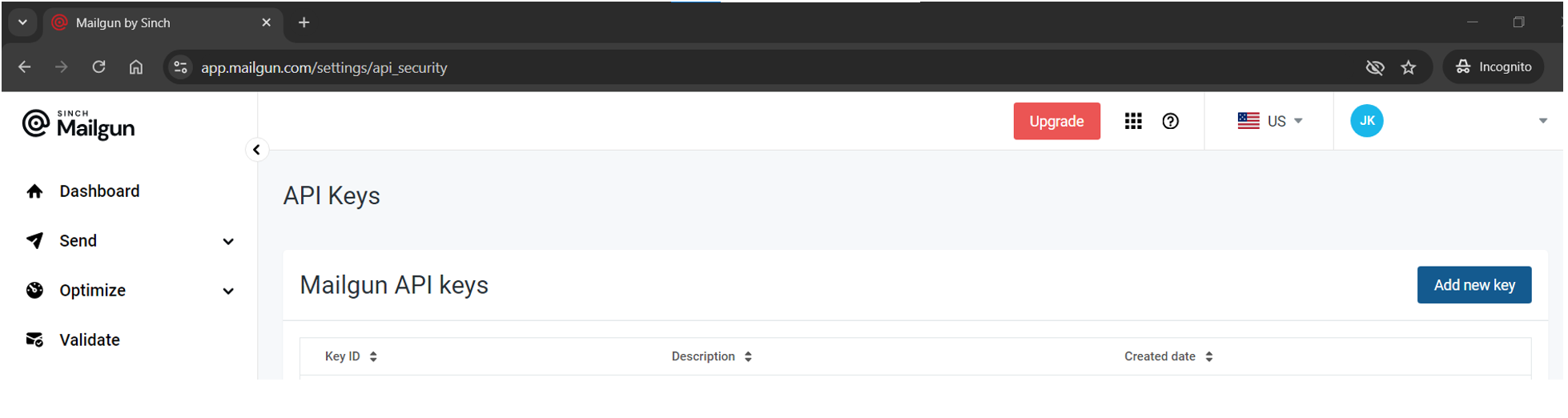
Type in a description (such as "Bulk Email Validation API Key") and click Create Key:
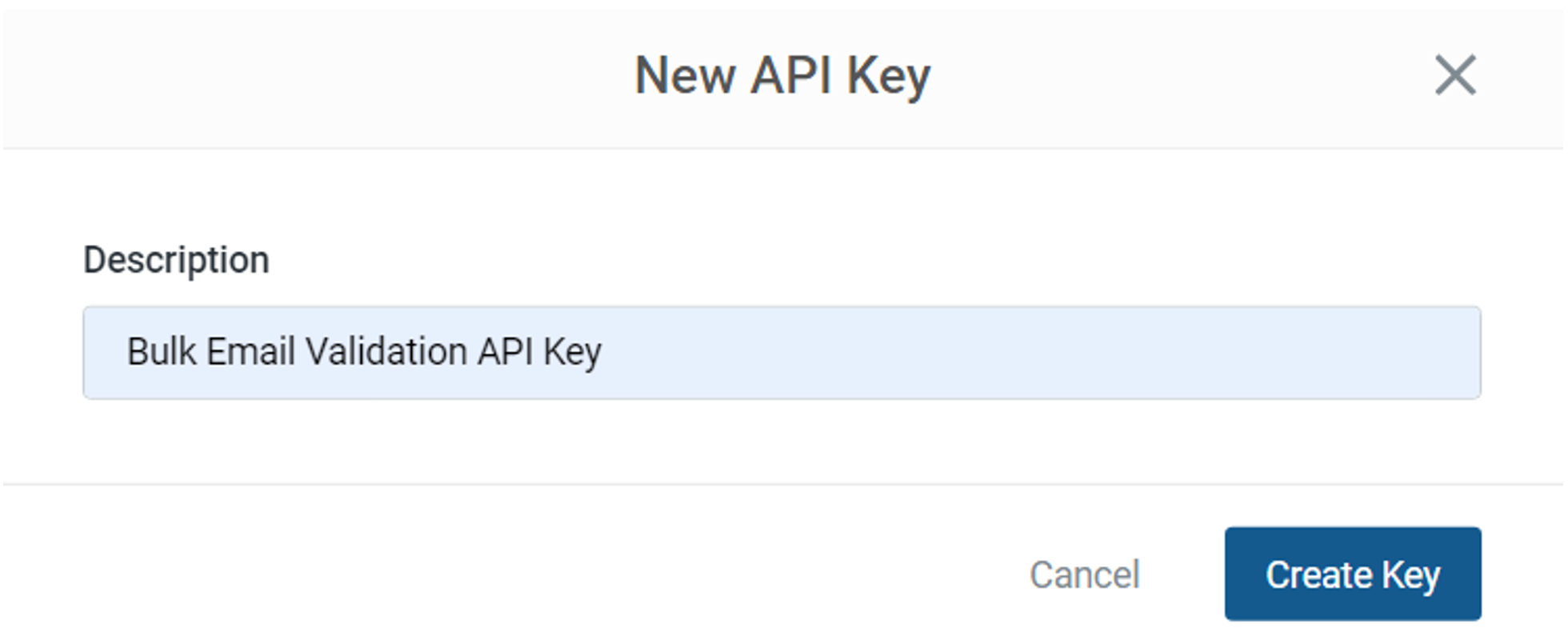
Once the API key is created, copy the key information, as you'll need it later on. If you forget to copy the information or lose the key, don't worry; you can delete your API key and create a new one.
Setting up a project directory and dependencies
Create a project directory on your machine and switch to that directory. Next, open a terminal with a path set to the current project directory and execute the following command to create a Python virtual environment for this tutorial:
python -m venv venv
Activate the virtual environment:
venv\Scripts\activate
Then, install the requests library, which will be used to interact with Mailgun endpoints:
pip install requests
Creating a Python project and configuration script
Once you've activated your virtual environment, create a new directory inside the project directory named standalone_python_scripts
, where you'll develop the Python modules required to carry out bulk email validation using Mailgun endpoints.
In this new directory, create a new Python file named config.py
and paste in the following code to configure the standalone Python script application:
This config module holds the configuration variables required for carrying out the bulk email validation request.
Set up a new environment variable, MAILGUN_API_KEY, on your host machine by running the following command:
SET MAILGUN_API_KEY=<Your Mailgun API Key>
This assigns the API key that you generated earlier during Mailgun account setup.
If you're a Linux or Mac user, execute the following command:
export MAILGUN_API_KEY=<Your Mailgun API Key>
By storing the API key in an environment variable instead of hard-coding it in the config file, you keep sensitive information protected.
Leave the other variables' values as is for now. The LIST_NAME variable contains the identifier name for your mailing list to be validated. FILE_PATH contains the path to the input CSV file containing the list of emails to be validated.
Preparing your mailing list file
Now that the configuration script refers to a mailing list file path via the FILE_PATH variable, you need an actual mailing list with email addresses to validate. Create a file named mailing_list.csv
in the standalone_python_scripts directory
and paste in a few sample email addresses to start:
email
dummy_email@dummydomain.com
test_email@testemaildomain.com
non_existent_email_id_123456789@gmail.com
hellojohnsemail123@gmail.com
The email addresses used here are not valid. You can add more valid or invalid ones in the same format with your own desired list of email addresses.
Creating a bulk validation job
With the mailing list in place and the configuration for the validation process ready, it's time to set up a function to create a bulk email validation job with Mailgun.
In the same directory, create a Python script file named bulk_email_validation.py
and define a create_bulk_validation_job
function that takes two parameters, list_name
(name of the mailing list) and file_path
(path to the file containing emails):
The function sends a POST request to Mailgun's API to initiate the bulk email validation process. The request includes the file of email addresses that need to be validated.
Developing functions to check job status and download results
Now, write another function called get_bulk_validation_status
in the same bulk_email_validation.py
script that checks the status of the submitted validation job. The response includes a link to download the validation results in both CSV and JSON formats. In this tutorial, you'll use JSON to download and process the results further.
To download the validation results, the get_bulk_validation_status
function makes a call to another function named download_validation_results
. The downloaded result is placed inside the validation_results
directory in the form of a JSON file. Go ahead and create this directory inside the standalone_python_scripts
directory, then paste the following code defining these two functions into bulk_email_validation.py
:
The function sends a POST request to Mailgun's API to initiate the bulk email validation process. The request includes the file of email addresses that need to be validated.
Developing functions to check job status and download results
Now, write another function called get_bulk_validation_status
in the same bulk_email_validation.py
script that checks the status of the submitted validation job. The response includes a link to download the validation results in both CSV and JSON formats. In this tutorial, you'll use JSON to download and process the results further.
To download the validation results, the get_bulk_validation_status
function makes a call to another function named download_validation_results
. The downloaded result is placed inside the validation_results
directory in the form of a JSON file. Go ahead and create this directory inside the standalone_python_scripts
directory, then paste the following code defining these two functions into bulk_email_validation.py
:
Parsing and handling validation results
Once you've downloaded and gotten access to the validation results, you need to parse and process them. This step is crucial for identifying deliverable and undeliverable emails.
Paste the following code to define the function in bulk_email_validation.py
that does this described task:
Now, define the instructions to call these functions with the following code:
The final script
Overall, the entire bulk_email_validation.py
script does the heavy lifting of posting a bulk email validation request, querying the status of the submitted validation job request, and processing the results. Once you're done with all changes, your bulk_email_validation.py
script should look like this.
Throughout the script, the use of try...except blocks ensures any errors during the API calls are handled. This is especially important for production environments where unexpected issues can arise, like when your application is unable to reach the Mailgun service's endpoints or when you're trying to submit a validation job request with the same list name. The error messages and the comments in the source code help explain the various other scenarios that are handled.
If you're resubmitting the validation job request with the same list name (you'll learn how to execute the script in the following sections), the script is equipped to handle such scenarios as well by throwing the corresponding error message with a description:
2024-09-28 07:34:39,081 - ERROR - create_bulk_validation_job - Error creating validation job: 409 {"message":"List already exists."}
If you encounter a network error between your application and the Mailgun service, you can implement a retry mechanism. You can either create your own or use a library like tenacity to define how many times to retry the request if it fails due to temporary connectivity issues. You can also implement other error handling scenarios as your project or business demands.
At this point, you've finished setting up the project, and it's time to execute it.
Testing by submitting a bulk email validation job request
To execute the script to submit the bulk validation job request, open a terminal and switch to the standalone_python_scripts
directory. Execute the following command:
python bulk_email_validation.py
You should see an output indicating that the job request has been successfully submitted to the Mailgun service:
2024-09-28 19:47:34,012 - INFO - create_bulk_validation_job - Bulk validation job created successfully for bulk_mailing_list_validation_1
Testing by fetching the status of the bulk email validation job
At times, based on the volume of email addresses to be validated, the submitted job will take varying times to complete. To get the status of the job request and to process the results if the request is complete, edit the config.py code to update the COMMAND configuration to the value get_job_status
. Once done, execute the following command to carry out this task:
python bulk_email_validation.py
You should see an output indicating that the validation job is complete, the results are downloaded, and then processed:
2024-09-28 19:48:07,723 - INFO - get_bulk_validation_status - Successfully retrieved validation status for bulk_mailing_list_validation_1
2024-09-28 19:48:08,375 - INFO - download_validation_results - Successfully downloaded validation results.
2024-09-28 19:48:08,378 - INFO - process_validation_results - Total email addresses validated: 191
2024-09-28 19:48:08,378 - INFO - process_validation_results - Found 178 deliverable emails
2024-09-28 19:48:08,378 - WARNING - process_validation_results - Found some emails that need to be verified because of its risky or undeliverable state.
2024-09-28 19:48:08,378 - WARNING - process_validation_results - Total emails to be verified: 13
2024-09-28 19:48:08,378 - WARNING - process_validation_results - Emails to be verified:
dummy_email@dummydomain1.com, dummy_email@dummydomain2.com, hellojohnsemail123@gmail.com, dummy_email@dummydomain3.com, non_existent_email_id_123456789@gmail.com, dummy_email@dummydomain.com, dummy_email@dummydomain4.com, dummy_email@dummydomain5.com, dummy_email@dummydomain6.com, dummy_email@dummydomain7.com, dummy_email@dummydomain8.com, test_email@testemaildomain.com, electronix84@gmail.com
This output indicates that out of 191 email addresses submitted for validation, 178 are categorized as deliverable, and the remaining 13 are not.
The script identifies the email addresses to be verified by parsing the response from Mailgun APIs and displaying the list in the output. It's now up to you to decide what course of action to take based on your project or business needs.
Integrating bulk email validation in a Django application
Now that you've learned these techniques, you can opt to apply them in your Django application by creating endpoints to upload a mailing list with bulk email addresses and validating them.
You can refer to or clone this GitHub repository if you want to experiment and follow along. The bulk_email_validation directory holds the Django project's source code. The following are the key files involved in this project:
The requirements.txt file contains the dependencies required for this Django project.
The config.py file holds the configuration related to the Mailgun service.
The views.py file contains the logic to process the request and responses related to bulk email validation endpoints.
The serializers.py file contains classes that help translate data between Python objects and formats like JSON, making it easy to send or receive data for bulk email validation APIs with just a few lines of code.
The urls.py file contains the two endpoints related to bulk email validation. One endpoint is for submitting the bulk email validation job request; the other is for getting the status and processing the results.
The screenshots below are intended to help you understand the endpoints that this Django application deals with and the output it can generate.
The first screenshot indicates the POST request with options to upload the mailing list file and set a mailing list name, along with the response received after the successful submission of the bulk email validation job request:
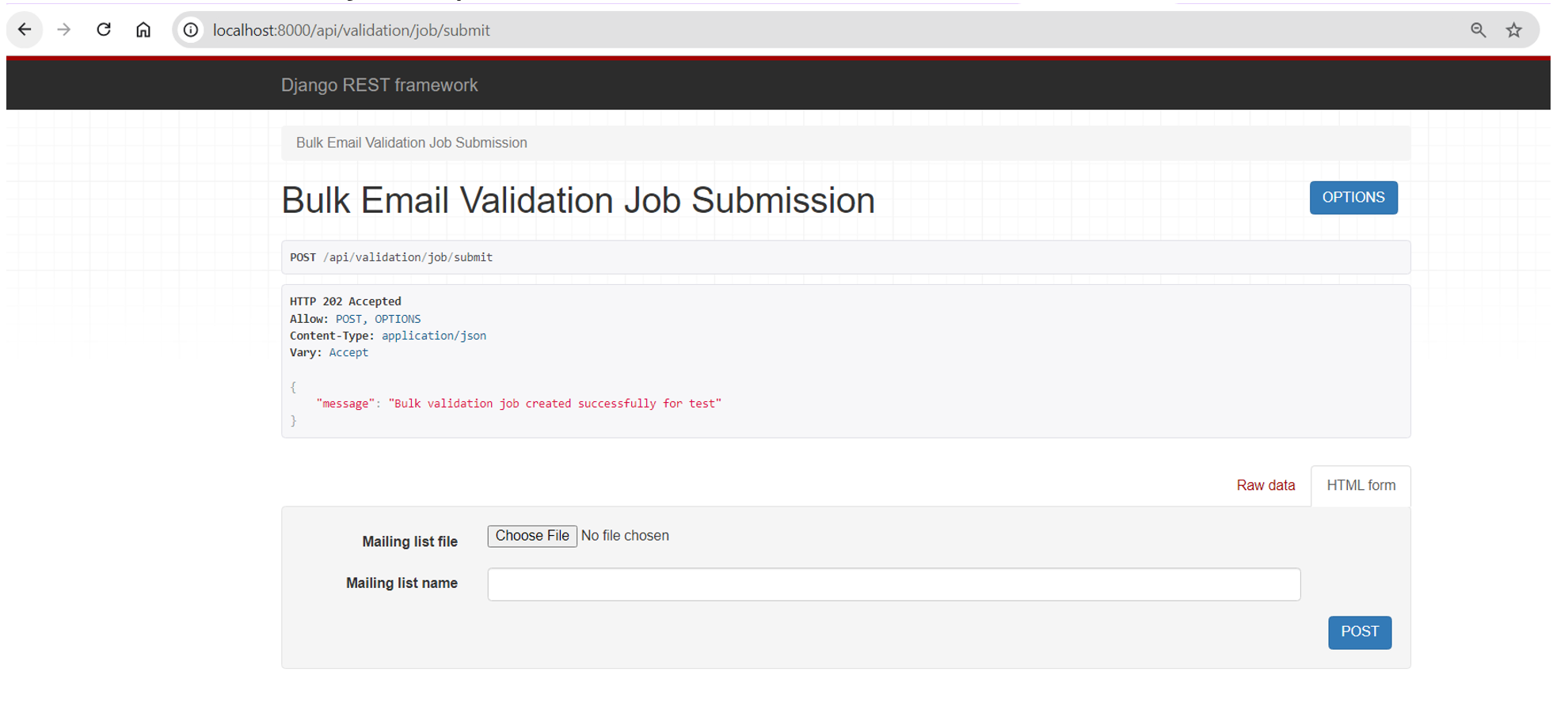
The second screenshot indicates the successful retrieval of the validation job response after its completion:
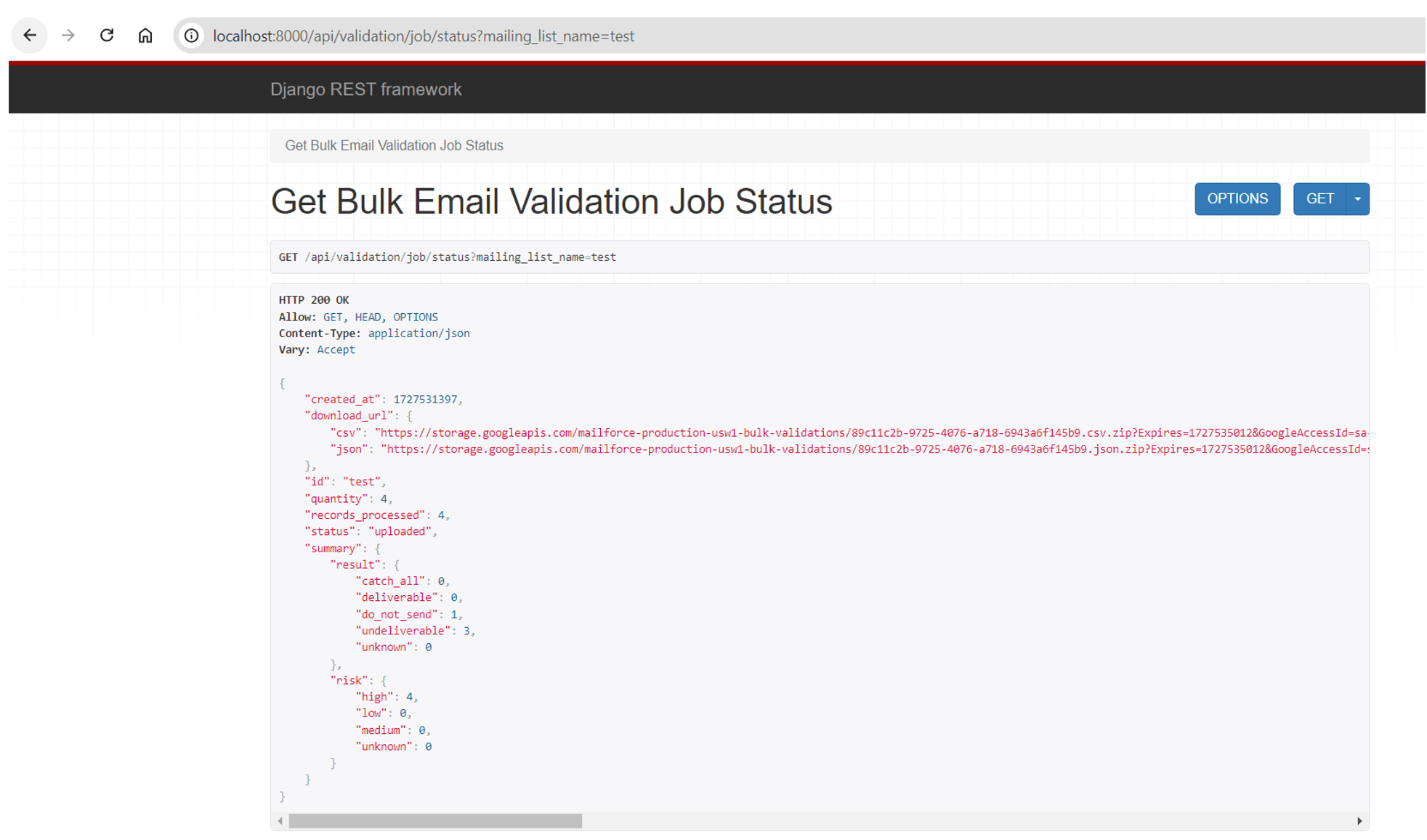
You can have a frontend application developed with any frontend technology that you wish and call these Django application endpoints to meet your bulk email validation goal.
Wrapping up
This tutorial taught you how to integrate Mailgun's Bulk Email Validation API into your Python applications to ensure the accuracy and deliverability of your email lists. From setting up your Mailgun account, obtaining the API key, and securely handling it, to implementing bulk validation, sending POST and GET requests, and processing the results, you now know how to automate the email verification process. You also explored how to manage invalid emails, handle common errors, and integrate email validation into larger web applications like Django. The entire source code presented for this tutorial is available in this GitHub repo.
By following these steps, you can effectively identify deliverable emails from your large mailing list, thus improving the quality of your email campaigns, reducing bounce rates, and safeguarding your sender reputation. Using Mailgun's powerful Bulk Email Validation API not only streamlines this process but also ensures your email delivery is more reliable and efficient. As you continue to optimize your email strategy, check out Mailgun, explore its various product offerings, and utilize them to further enhance your email campaigns and maximize their impact.
Get expert advice
Deliverability does require a certain level of competency. If you need guidance navigating the complexities of inbox placement, ask about Sinch Mailgun’s Deliverability Services. We provide dedicated technical experts, regular reporting, custom deliverability strategies, and access to helpful tools.