How to send emails in JavaScript: Frontend and backend
Adding email functionality to your web application helps you keep users engaged and informed. Whether its account verification emails, or password reset links, email keeps communication direct and reliable. In this post, learn how to send these emails with both a backend and frontend approach using JavaScript.
PUBLISHED ON
There are two ways to integrate email into your app: a frontend-only approach or a backend-powered method. The frontend approach uses the mailto
protocol and vanilla JavaScript, but it’s limited and exposes sensitive information. The backend method, using Node.js
and the Mailgun API, gives you better control, security, and flexibility.
This guide walks you through both options, so you can decide what works best for your setup.
Table of contents
Sending emails from the frontend using the mailto protocol
Set up your development environment
Retrieve your Mailgun API key
Send emails using Mailgun's Node.js SDK
Best practices for sending emails from the backend
Sending emails from the frontend
The Simple Mail Transfer Protocol (SMTP) is the standard protocol for sending emails on the internet. To send an email from the frontend, you need a connection to an SMTP server, which requires a username, password, or API key provided by an SMTP host like Mailgun for authentication. However, that connection will also expose the SMTP host's credentials, leaving them vulnerable to theft.
As a more secure alternative, you can set up a dedicated serverless function or a simple backend that uses the Mailgun API to send emails and connects securely with your frontend. However, this approach is not "frontend-only," as it involves servers and can be challenging to set up without a traditional backend.
The most feasible and secure "frontend-only" method for sending emails involves using a hyperlink to trigger the user's default mail app with a prefilled template for them to send. This approach doesn't need an SMTP server or credentials because the email is sent through the user's email client instead of your application. You can set this up using the mailto protocol.
Sending emails from the frontend using the mailto protocol
The mailto
protocol is a URL scheme that lets users send emails by clicking a link. When a user clicks a mailto
link, it opens their default mailbox with the recipient of the email prefilled. In addition to the recipient of the email being prefilled, you can also customize your mailto
link to include a subject, a body, and carbon copy (cc) and blind carbon copy (bcc).
mailto links use the following syntax:
mailto:sAddress[sHeaders]
sAddress
refers to one or more valid email addresses separated by a semicolon, and sHeaders
(optional) refers to query parameters containing the subject, body, cc, and bcc.
To send an email from your frontend using the mailto
protocol, create an HTML form that allows users to input the recipient(s), subject, body, cc, and bcc. You can use these inputs to generate a mailto
URL with JavaScript. When the user submits the form, the browser opens the user's mailbox using the mailto
link you created.
To create the form, add the following code block to a new HTML file:
The form includes a recipient, a cc, a bcc, a subject, a body field, and a submit button. The code also adds some basic styling to the form:
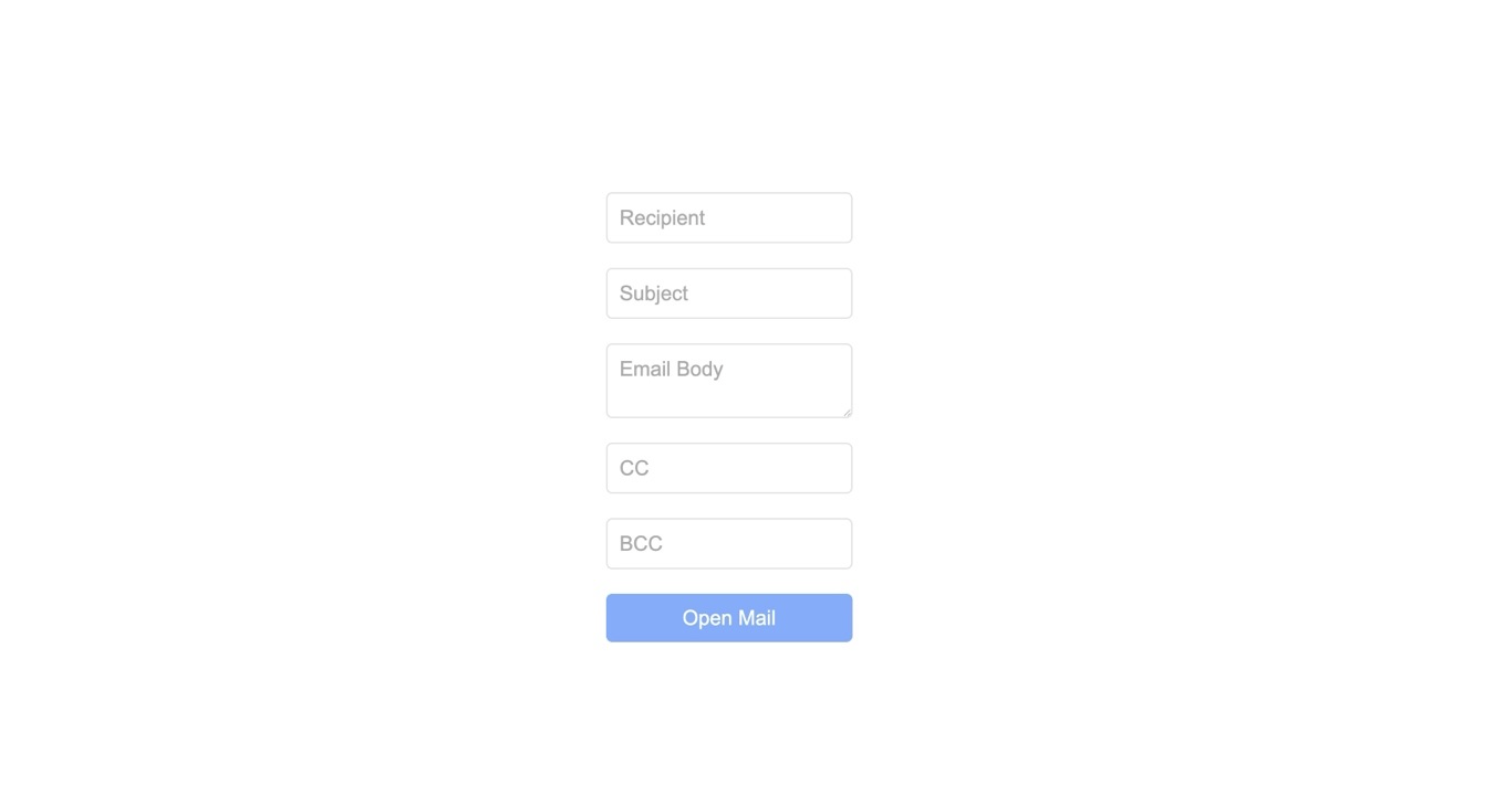
Create a script.js
file and paste in the following code to add a click
event listener to the submit button, which triggers the openMailBox
function:
The openMailBox
function extracts the form's values and creates a mailto link by concatenating the encoded version of the values to avoid issues with special characters (spaces, &, =, ?
) in the mailto URL. It also opens the mailto
link in a new tab to trigger the user's mailbox.
This method of sending emails is useful for small-scale applications and websites where an email backend is unnecessary (such as "Contact Us" links, feedback forms, and support tickets). However, it has some significant disadvantages, including the risk of exposing credentials, a lack of email delivery tracking, and challenges with scaling.
Sending emails from the backend
For more complex or sensitive email handling scenarios, sending emails from a backend offers enhanced security as credentials are stored on the server. It's also more reliable as backend systems can manage bulk emails and email queues, ensuring messages are sent reliably even during high traffic.
You can use a mailing service like Mailgun to simplify this process. Mailgun handles the complexities of email protocols (SMTP, HTTP APIs), domain verification, and spam prevention.
Set up your development environment
To send emails using Node.js and Mailgun, you'll first need to create a development environment by running the following command:
mkdir email-service && cd email-service && npm init -y
The command above creates an email-service
folder and initializes npm with its defaults in the folder.
Install the required packages with the following command:
npm install mailgun.js form-data dotenv
The installed dependencies include:
Mailgun.js
(Mailgun's Node.js SDK), which you need to interact with Mailgun using Node.js.Dotenv
, a library that loads environmental variables toprocess.env
. You'll need it to manage your sensitive credentials securely.Form-Data
, a library that creates readablemultipart/form-data
streams. Mailgun only accepts data inmultipart/form-data
, so you need this library to initialize the SDK.
After installing the dependencies above, you need to get an API key from your Mailgun dashboard.
Retrieve your Mailgun API key
To get your API key, first log in to your Mailgun account. On your user dashboard, click the dropdown button at the top right of your screen and click the API Security button.
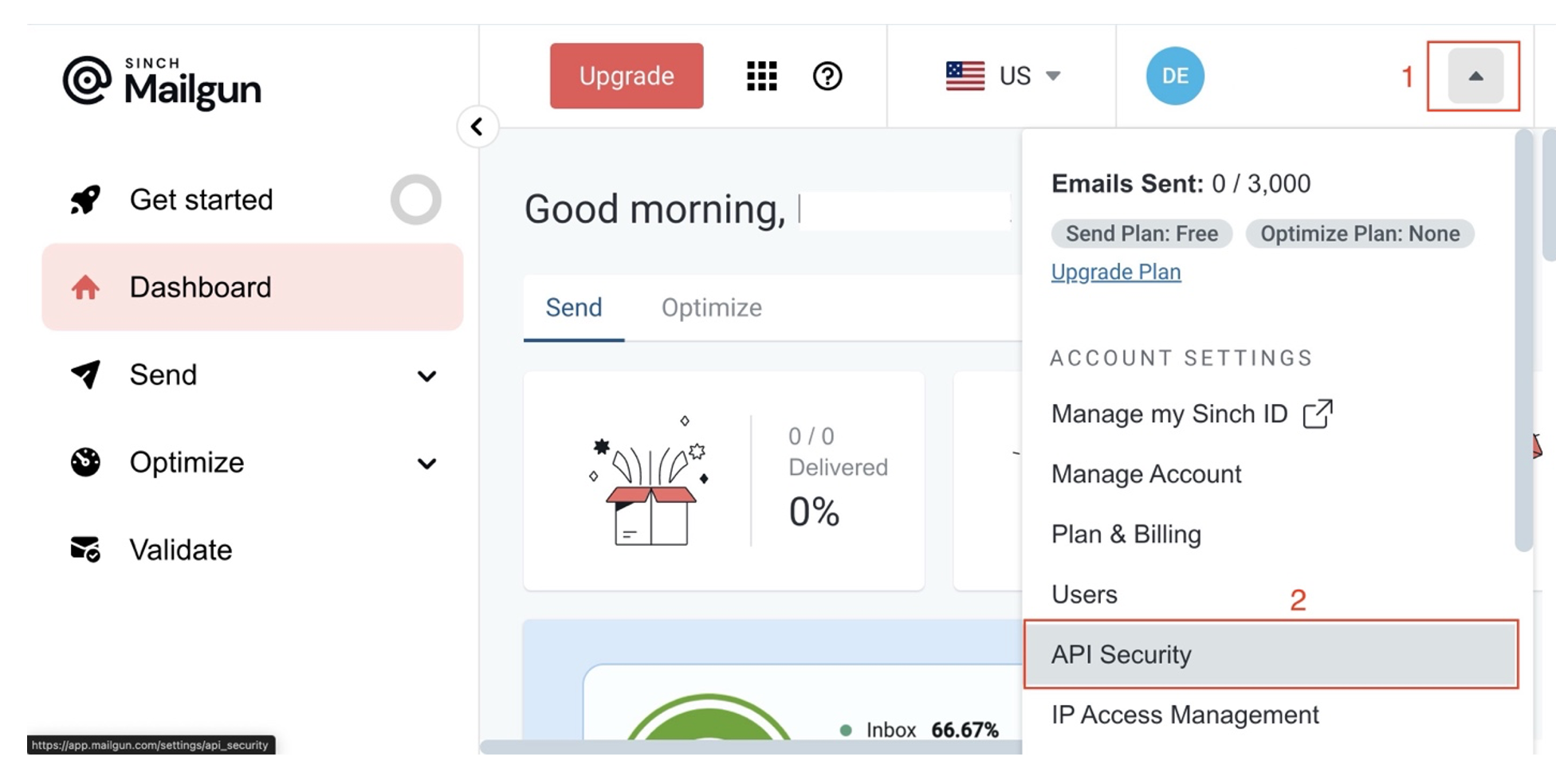
On the API Security page, click the Add new key button to reveal a modal with a form. Fill out the description on the pop-up modal and click the Create Key button, copy the API key displayed, and add it to your .env
file.
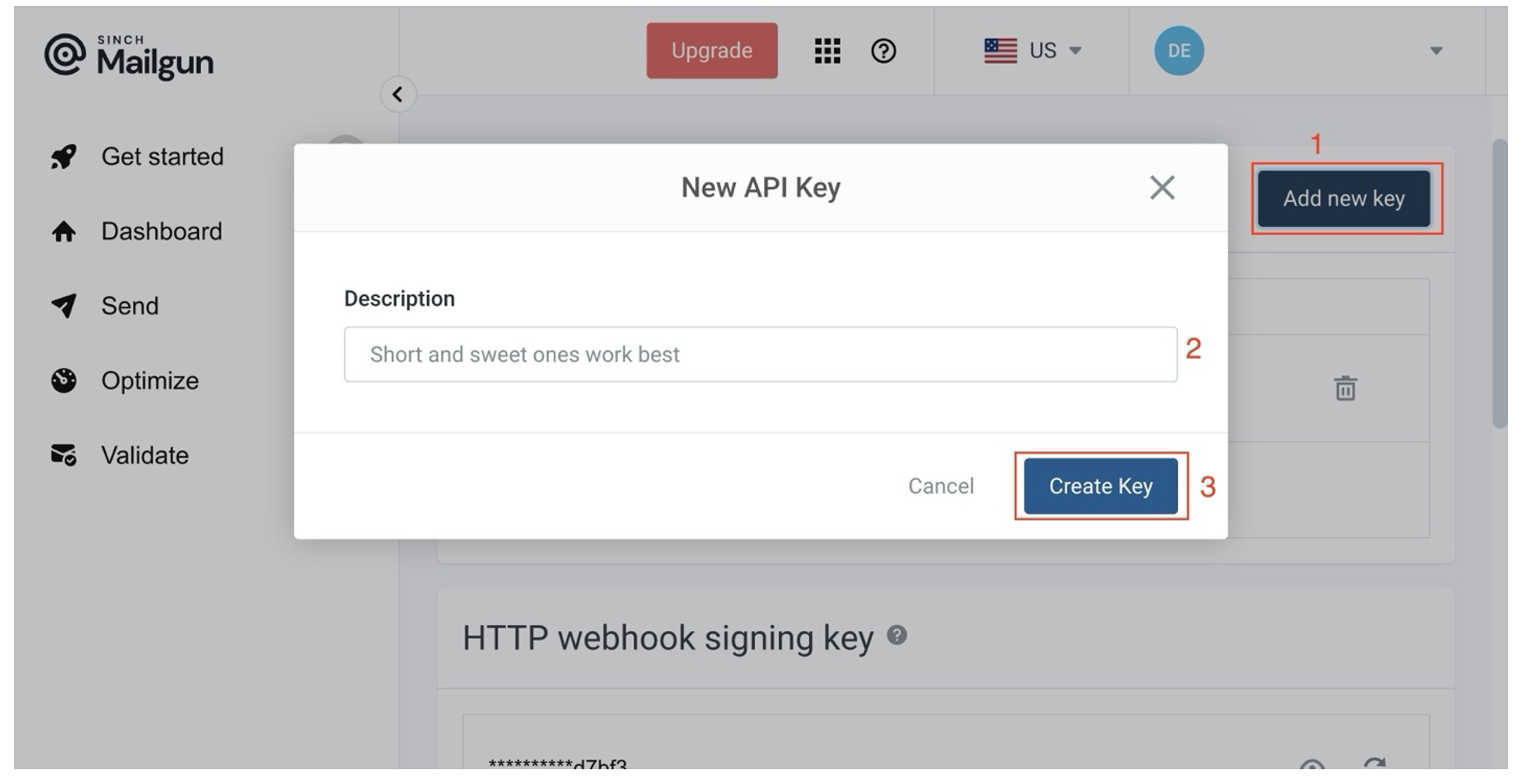
Next, create an index.js
file and a .env
file in your project directory. In your .env
file, add the variables below, and replace the placeholder value with its actual value:
MAILGUN_API_KEY = <API_KEY>
Send emails using Mailgun's Node.js SDK
Now that you have your API key, you can initialize your Mailgun SDK. In your index.js
file, add the required imports and initialize dotenv
by adding the following code:
You can send emails using the Mailgun SDK's messages.create
function. It takes an email domain and an object containing details about the email you want to send as arguments. The email domain must be added to your Mailgun account and verified. Follow this guide to add and verify a custom email domain. Alternatively, you can use the default sandbox domain provided by Mailgun.
The details in the object include (but are not limited to) the following:
from
shows the recipient who the email is from. This property should be your registered domain.to
is the recipient's (or recipients') email address(es).subject
is the subject of the email.text
is the content of the email.html
is the content of the email in HTML. One function call cannot havetext
andhtml
properties at the same time; one would be discarded.
You can explore more of the available options in the official Node.js SDK docs.
For example, add the code block below to your index.js
file to implement a function that sends an email:
You can call the function like this:
Execute the function by running the following command:
node index
A response similar to the following should be logged to the console:
{
status: 200,
id: '<2025011324959830.5ae661a4e9fbf66f@sandboxca2ef9affjfiokfi7e431e95fc6ec3.mailgun.org>',
message: 'Queued. Thank you.'
}
The email should now be delivered to the recipient's mailbox.
Best practices for sending emails from the backend
Without proper setup, emails sent from your backend may be flagged as spam, fail to reach recipients, or get blocked by providers. To improve deliverability, follow these best practices:
Use a reputable ESP: When sending emails from the backend, it's important to use a reputable email service provider (ESP) like Mailgun to handle the complexities of email delivery, including ensuring your emails are not marked as spam by enforcing email domain authentication (SPF, DKIM, DMARC).
Store API keys securely: Another important consideration is securely storing your API keys using environment variables to ensure they're not stolen and used for malicious purposes, thus affecting your sender reputation. Mailgun offers scoped API keys, allowing you to grant specific permissions (such as "sending only") to minimize the risk of abuse.
Monitor email analytics: You also need to track delivery rates, bounces, and failed emails in order to take corrective action. Mailgun's dashboard provides insights into bounce rates, delivery rates, and engagement metrics that help you optimize email campaigns. Mailgun also offers an API to validate email addresses, ensuring you only send emails to valid recipients, thus reducing your bounce rates.
Following these best practices will help ensure reliable email delivery and minimize spam filtering.
Wrapping up
In this article, you explored how to send emails using a frontend-only approach and a backend approach. The frontend-only approach is simple to set up, but it's not ideal for complex email functions due to its limitations. The backend approach offers a more feature-rich solution to email integration that's made simpler by email service providers like Mailgun.
With Mailgun, you can send emails securely, optimize your email deliverability, and manage and validate your mailing lists.